In this article, I am going to show you how to install Deno and write a simple Deno program and execute it, from scratch. We will be developing basic deno http server which will listen to a predefined port and respond. Let’s get started.
Installing Deno on Windows:
Open Windows Power Shell and run the following command –
iwr https://deno.land/x/install/install.ps1 -useb | iex
If you are Mac or Linux user, in the shell execute the following command –
curl -fsSL https://deno.land/x/install/install.sh | sh
This will install Deno in your machine, you will see the following output in the power shell window-
PS C:\Users> iwr https://deno.land/x/install/install.ps1 -useb | iex
Deno was installed successfully to C:\Users\Lok\.deno\bin\deno.exe
Run 'deno --help' to get started
Now check the installed Deno version by running deno --version
command. If it is installed correctly, it will output the version as shown below-
PS C:\Users> deno --version
deno 1.4.2
v8 8.7.75
typescript 4.0.3

Now, let’s try running a sample Deno program. Run the following command on Windows Power Shell.
PS C:\Users> deno run https://deno.land/std/examples/welcome.ts
It will output Welcome to Deno as shown below-
PS C:\Users> deno run https://deno.land/std/examples/welcome.ts
←[0m←[32mDownload←[0m https://deno.land/std/examples/welcome.ts
←[0m←[33mWarning←[0m Implicitly using latest version (0.71.0) for https://deno.land/std/examples/welcome.ts
←[0m←[32mDownload←[0m https://deno.land/[email protected]/examples/welcome.ts
←[0m←[32mCheck←[0m https://deno.land/[email protected]/examples/welcome.ts
Welcome to Deno 🦕
Creating Simple Deno Server:
Now, let us create a simple Deno server app. Create a directory deno-app, add a new file say, server.ts and write the following code.
//server.ts
import { serve } from "https://deno.land/[email protected]/http/server.ts";
const s = serve({ port: 8000 });
console.log("http://localhost:8000/");
for await (const req of s) {
req.respond({ body: "Hello world, this is my first Deno app! " });
}
Execute the code by running deno run --allow-net server.ts
command-
PS E:\Deno\deno-app> deno run --allow-net server.ts
←[0m←[32mCheck←[0m file:///E:/Deno/deno-app/server.ts
http://localhost:8000/

That’s all. Now, our Deno server is up and it is listening to port 8000. You can open a browser and browse the URL http://localhost:8000/
, you will see the following output-
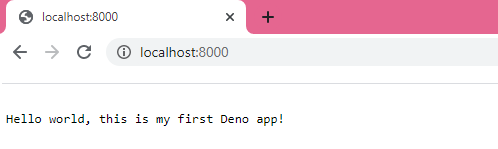
Great! We have successfully developed a basic http server using Deno. As you see, whatever you type after localhost:8000, it will always return same Hello world message, because we have not written any code to handle different requests. In the upcoming article, I will show you how to handle different requests and respond back with data.
List of Permission flag
As deno is secure by default it doesn’t have any permission enable. These flags will be useful while for permission while running a file.
— allow-env:
Allow environment access.— allow-hrtime:
Allow high-resolution time measurement.— allow-net:
Allow network access.— allow-plugin:
Allow loading plugins.— allow-read:
Allow file system read access.— allow-run:
Allow running subprocesses.— allow-write:
Allow file system write access.— allow-all:
: Give all access.
Hope this helps. Thanks for reading.
Halfy
←[0m←[32m
Why this is show in terminal?
Datatype
Yeh, that is timestamp displayed in terminal. Nothing to worry about it.